
[ad_1]
Improvement of Ethereum has been progressing more and more rapidly this previous month. The discharge of PoC5 (“proof of idea 5”) final month the day earlier than the sale marked an necessary occasion for the undertaking, as for the primary time we had two purchasers, one written in C++ and one in Go, completely interoperating with one another and processing the identical blockchain. Two weeks later, the Python consumer was additionally added to the checklist, and now a Java model can be virtually performed. At the moment, we’re within the means of utilizing an preliminary amount of funds that we’ve got already withdrawn from the Ethereum exodus deal with to broaden our operations, and we’re laborious at work implementing PoC6, the following model within the sequence, which options quite a lot of enhancements.
At this level, Ethereum is at a state roughly just like Bitcoin in mid-2009; the purchasers and protocol work, and other people can ship transactions and construct decentralized functions with contracts and even fairly person interfaces inside HTML and Javascript, however the software program is inefficient, the UI underdeveloped, networking-level inefficiencies and vulnerabilities will take some time to get rooted out, and there’s a very excessive danger of safety holes and consensus failures. With the intention to be snug releasing Ethereum 1.0, there are solely 4 issues that completely have to be performed: protocol and network-level safety testing, digital machine effectivity upgrades, a really massive battery of assessments to make sure inter-client compatibility, and a finalized consensus algorithm. All of those are actually excessive on our precedence checklist; however on the identical time we’re additionally working in parallel on highly effective and easy-to-use instruments for constructing decentralized functions, contract normal libraries, higher person interfaces, mild purchasers, and all the different small options that push the event expertise from good to greatest.
PoC6
The foremost modifications which are scheduled for PoC6 are as follows:
- The block time is decreased from 60 seconds to 12 seconds, utilizing a brand new GHOST-based protocol that expands upon our earlier efforts at decreasing the block time to 60 seconds
- The ADDMOD and MULMOD (unsigned modular addition and unsigned modular multiplication) are added at slots 0x14 and 0x15, respectively. The aim of those is to make it simpler to implement sure sorts of number-theoretic cryptographic algorithms, eg. elliptic curve signature verification. See right here for some instance code that makes use of these operations.
- The opcodes DUP and SWAP are faraway from their present slots. As an alternative, we’ve got the brand new opcodes DUP1, DUP2 … DUP16 at positions 0x80 … 0x8f and equally SWAP1 … SWAP16 at positions 0x90 … 0x9f. DUPn copies the nth highest worth within the stack to the highest of the stack, and SWAPn swaps the best and (n+1)-th highest worth on the stack.
- The with assertion is added to Serpent, as a guide approach of utilizing these opcodes to extra effectively entry variables. Instance utilization is discovered right here. Word that that is a sophisticated characteristic, and has a limitation: should you stack so many layers of nesting beneath a with assertion that you find yourself attempting to entry a variable greater than 16 stack ranges deep, compilation will fail. Ultimately, the hope is that the Serpent compiler will intelligently select between stack-based variables and memory-based variables as wanted to maximise effectivity.
- The POST opcode is added at slot 0xf3. POST is just like CALL, besides that (1) the opcode has 5 inputs and 0 outputs (ie. it doesn’t return something), and (2) the execution occurs asynchronously, after every thing else is completed. Extra exactly, the method of transaction execution now includes (1) initializing a “put up queue” with the message embedded within the transaction, (2) repeatedly processing the primary message within the put up queue till the put up queue is empty, and (3) refunding fuel to the transaction origin and processing suicides. POST provides a message to the put up queue.
- The hash of a block is now the hash of the header, and never all the block (which is the way it actually ought to have been all alongside), the code hash for accounts with no code is “” as an alternative of sha3(“”) (making all non-contract accounts 32 bytes extra environment friendly), and the to deal with for contract creation transactions is now the empty string as an alternative of twenty zero bytes.
On Effectivity
Except for these modifications, the one main concept that we’re starting to develop is the idea of “native contract extensions”. The thought comes from lengthy inside and exterior discussions in regards to the tradeoffs between having a extra lowered instruction set (“RISC“) in our digital machine, restricted to primary reminiscence, storage and blockchain interplay, sub-calls and arithmetic, and a extra complicated instruction set (“CISC“), together with options resembling elliptic curve signature verification, a wider library of hash algorithms, bloom filters, and knowledge constructions resembling heaps. The argument in favor of the lowered instruction set is twofold. First, it makes the digital machine easier, permitting for simpler improvement of a number of implementations and decreasing the chance of safety points and consensus failures. Second, no particular set of opcodes will ever embody every thing that folks will wish to do, so a extra generalized answer can be rather more future-proof.
The argument in favor of getting extra opcodes is easy effectivity. For example, think about the heap). A heap is a knowledge construction which helps three operations: including a price to the heap, rapidly checking the present smallest worth on the heap, and eradicating the smallest worth from the heap. Heaps are notably helpful when constructing decentralized markets; the best method to design a market is to have a heap of promote orders, an inverted (ie. highest-first) heap of purchase orders, and repeatedly pop the highest purchase and promote orders off the heap and match them with one another whereas the ask worth is larger than the bid. The best way to do that comparatively rapidly, in logarithmic time for including and eradicating and fixed time for checking, is utilizing a tree:
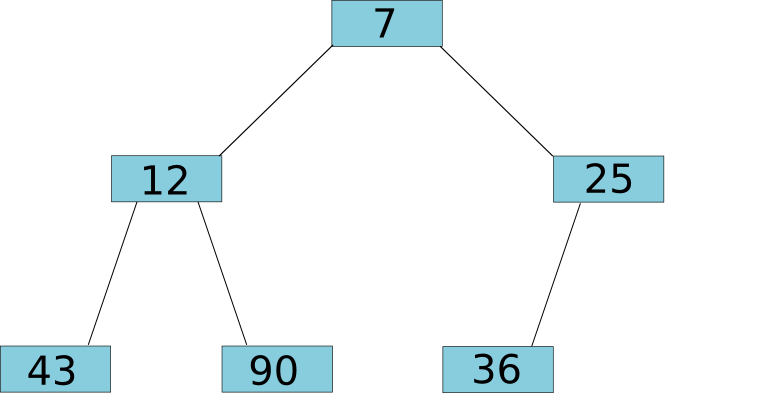
The important thing invariant is that the father or mother node of a tree is all the time decrease than each of its kids. The best way so as to add a price to the tree is so as to add it to the top of the underside degree (or the beginning of a brand new backside degree if the present backside degree is full), after which to maneuver the node up the tree, swapping it with its dad and mom, for so long as the father or mother is larger than the kid. On the finish of the method, the invariant is once more glad with the brand new node being within the tree on the proper place:
To take away a node, we pop off the node on the high, take a node out from the underside degree and transfer it into its place, after which transfer that node down the tree as deep as is sensible:
And to see what the bottom node is, we, nicely, take a look at the highest. The important thing level right here is that each of those operations are logarithmic within the variety of nodes within the tree; even when your heap has a billion gadgets, it takes solely 30 steps so as to add or take away a node. It is a nontrivial train in pc science, however should you’re used to coping with timber it isn’t notably sophisticated. Now, let’s attempt to implement this in Ethereum code. The complete code pattern for that is right here; for these the father or mother listing additionally comprises a batched market implementation utilizing these heaps and an try at implementing futarchy utilizing the markets. Here’s a code pattern for the a part of the heap algorithm that handles including new values:
# push if msg.knowledge[0] == 0: sz = contract.storage[0] contract.storage[sz + 1] = msg.knowledge[1] ok = sz + 1 whereas ok > 1: backside = contract.storage[k] high = contract.storage[k/2] if backside < high: contract.storage[k] = high contract.storage[k/2] = backside ok /= 2 else: ok = 0 contract.storage[0] = sz + 1
The mannequin that we use is that contract.storage[0] shops the dimensions (ie. variety of values) of the heap, contract.storage[1] is the basis node, and from there for any n <= contract.storage[0], contract.storage[n] is a node with father or mother contract.storage[n/2] and youngsters contract.storage[n*2] and contract.storage[n*2+1] (if n*2 and n*2+1 are lower than or equal to the heap measurement, after all). Comparatively easy.
Now, what’s the issue? Briefly, as we already talked about, the first concern is inefficiency. Theoretically, all tree-based algorithms have most of their operations take log(n) time. Right here, nevertheless, the issue is that what we even have is a tree (the heap) on high of a tree (the Ethereum Patricia tree storing the state) on high of a tree (leveldb). Therefore, the market designed right here really has log3(n) overhead in follow, a slightly substantial slowdown.
As one other instance, during the last a number of days I’ve written, profiled and examined Serpent code for elliptic curve signature verification. The code is principally a reasonably easy port of pybitcointools, albeit some makes use of of recursion have been changed with loops as a way to enhance effectivity. Even nonetheless, the fuel value is staggering: a median of about 340000 for one signature verification.
And this, thoughts you, is after including some optimizations. For instance, see the code for taking modular exponents:
with b = msg.knowledge[0]: with e = msg.knowledge[1]: with m = msg.knowledge[2]: with o = 1: with bit = 2 ^ 255: whereas gt(bit, 0): # A contact of loop unrolling for 20% effectivity achieve o = mulmod(mulmod(o, o, m), b ^ !(!(e & bit)), m) o = mulmod(mulmod(o, o, m), b ^ !(!(e & div(bit, 2))), m) o = mulmod(mulmod(o, o, m), b ^ !(!(e & div(bit, 4))), m) o = mulmod(mulmod(o, o, m), b ^ !(!(e & div(bit, 8))), m) bit = div(bit, 16) return(o)
This takes up 5084 fuel for any enter. It’s nonetheless a reasonably easy algorithm; a extra superior implementation could possibly pace this up by as much as 50%, however even nonetheless iterating over 256 bits is pricey it doesn’t matter what you do.
What these two examples present is that high-performance, high-volume decentralized functions are in some circumstances going to be fairly troublesome to write down on high of Ethereum with out both complicated directions to implement heaps, signature verification, and so forth within the protocol, or one thing to switch them. The mechanism that we are actually engaged on is an try conceived by our lead developer Gavin Wooden to basically get the most effective of each worlds, preserving the generality of straightforward directions however on the identical time getting the pace of natively applied operations: native code extensions.
Native Code Extensions
The best way that native code extensions work is as follows. Suppose that there exists some operation or knowledge construction that we wish Ethereum contracts to have entry to, however which we are able to optimize by writing an implementation in C++ or machine code. What we do is we first write an implementation in Ethereum digital machine code, take a look at it and ensure it really works, and publish that implementation as a contract. We then both write or discover an implementation that handles this job natively, and add a line of code to the message execution engine which appears to be like for calls to the contract that we created, and as an alternative of sub-calling the digital machine calls the native extension as an alternative. Therefore, as an alternative of it taking 22 seconds to run the elliptic curve restoration operation, it might take solely 0.02 seconds.
The issue is, how can we guarantee that the charges on these native extensions should not prohibitive? That is the place it will get tough. First, let’s make a couple of simplifications, and see the place the financial evaluation leads. Suppose that miners have entry to a magic oracle that tells them the utmost period of time {that a} given contract can take. With out native extensions, this magic oracle exists now – it consists merely of trying on the STARTGAS of the transaction – however it turns into not fairly so easy when you might have a contract whose STARTGAS is 1000000 and which appears to be like like it might or might not name a couple of native extensions to hurry issues up drastically. However suppose that it exists.
Now, suppose {that a} person is available in with a transaction spending 1500 fuel on miscellaneous enterprise logic and 340000 fuel on an optimized elliptic curve operation, which really prices solely the equal of 500 fuel of regular execution to compute. Suppose that the usual market-rate transaction payment is 1 szabo (ie. micro-ether) per fuel. The person units a GASPRICE of 0.01 szabo, successfully paying for 3415 fuel, as a result of he can be unwilling to pay for all the 341500 fuel for the transaction however he is aware of that miners can course of his transaction for 2000 fuel’ value of effort. The person sends the transaction, and a miner receives it. Now, there are going to be two circumstances:
- The miner has sufficient unconfirmed transactions in its mempool and is keen to expend the processing energy to supply a block the place the overall fuel used brushes in opposition to the block-level fuel restrict (this, to remind you, is 1.2 occasions the long-term exponential shifting common of the fuel utilized in latest blocks). On this case, the miner has a static quantity of fuel to replenish, so it desires the best GASPRICE it could actually get, so the transaction paying 0.01 szabo per fuel as an alternative of the market charge of 1 szabo per fuel will get unceremoniously discarded.
- Both not sufficient unconfirmed transactions exist, or the miner is small and never keen or in a position to course of each transaction. On this case, the dominating think about whether or not or not a transaction is accepted is the ratio of reward to processing time. Therefore, the miner’s incentives are completely aligned, and since this transaction has a 70% higher reward to value charge than most others it is going to be accepted.
What we see is that, given our magic oracle, such transactions can be accepted, however they’ll take a few additional blocks to get into the community. Over time, the block-level fuel restrict would rise as extra contract extensions are used, permitting the usage of much more of them. The first fear is that if such mechanisms turn into too prevalent, and the typical block’s fuel consumption can be greater than 99% native extensions, then the regulatory mechanism stopping massive miners from creating extraordinarily massive blocks as a denial-of-service assault on the community can be weakened – at a fuel restrict of 1000000000, a malicious miner might make an unoptimized contract that takes up that many computational steps, and freeze the community.
So altogether we’ve got two issues. One is the theoretical downside of the gaslimit turning into a weaker safeguard, and the opposite is the truth that we do not have a magic oracle. Fortuitously, we are able to remedy the second downside, and in doing so on the identical time restrict the impact of the primary downside. The naive answer is easy: as an alternative of GASPRICE being only one worth, there can be one default GASPRICE after which a listing of [address, gasprice] pairs for particular contracts. As quickly as execution enters an eligible contract, the digital machine would hold observe of how a lot fuel it used inside that scope, after which appropriately refund the transaction sender on the finish. To forestall fuel counts from getting too out of hand, the secondary fuel costs can be required to be not less than 1% (or another fraction) of the unique gasprice. The issue is that this mechanism is space-inefficient, taking on about 25 additional bytes per contract. A potential repair is to permit folks to register tables on the blockchain, after which merely seek advice from which payment desk they want to use. In any case, the precise mechanism shouldn’t be finalized; therefore, native extensions might find yourself ready till PoC7.
Mining
The opposite change that can doubtless start to be launched in PoC7 is a brand new mining algorithm. We (nicely, primarily Vlad Zamfir) have been slowly engaged on the mining algorithm in our mining repo, to the purpose the place there’s a working proof of idea, albeit extra analysis is required to proceed to enhance its ASIC resistance. The essential concept behind the algorithm is basically to randomly generate a brand new circuit each 1000 nonces; a tool able to processing this algorithm would have to be able to processing all circuits that may very well be generated, and theoretically there ought to exist some circuit that conceivably may very well be generated by our system that might be equal to SHA256, or BLAKE, or Keccak, or some other algorithms in X11. Therefore, such a tool must be a generalized pc – basically, the purpose is one thing that attempted to method mathematically provable specialization-resistance. With the intention to guarantee that all hash capabilities generated are safe, a SHA3 is all the time utilized on the finish.
After all, excellent specialization-resistance is unattainable; there’ll all the time be some options of a CPU that can show to be extraneous in such an algorithm, so a nonzero theoretical ASIC speedup is inevitable. At the moment, the largest risk to our method is probably going some type of quickly switching FPGA. Nevertheless, there’s an financial argument which exhibits that CPUs will survive even when ASICs have a speedup, so long as that speedup is low sufficient; see my earlier article on mining for an summary of a number of the particulars. A potential tradeoff that we should make is whether or not or to not make the algorithm memory-hard; ASIC resistance is difficult sufficient because it stands, and memory-hardness might or might not find yourself interfering with that purpose (cf. Peter Todd’s arguments that memory-based algorithms may very well encourage centralization); if the algorithm shouldn’t be memory-hard, then it might find yourself being GPU-friendly. On the identical time, we’re trying into hybrid-proof-of-stake scoring capabilities as a approach of augmenting PoW with additional safety, requiring 51% assaults to concurrently have a big financial part.
With the protocol in an more and more secure state, one other space wherein it’s time to begin growing is what we’re beginning to name “Ethereum 1.5” – mechanisms on high of Ethereum because it stands immediately, with out the necessity for any new changes to the core protocol, that enable for elevated scalability and effectivity for contracts and decentralized functions, both by cleverly combining and batching transactions or by utilizing the blockchain solely as a backup enforcement mechanism with solely the nodes that care a few specific contract operating that contract by default. There are a variety of mechanism on this class; that is one thing that can see significantly elevated consideration from each ourselves and hopefully others in the neighborhood.
[ad_2]